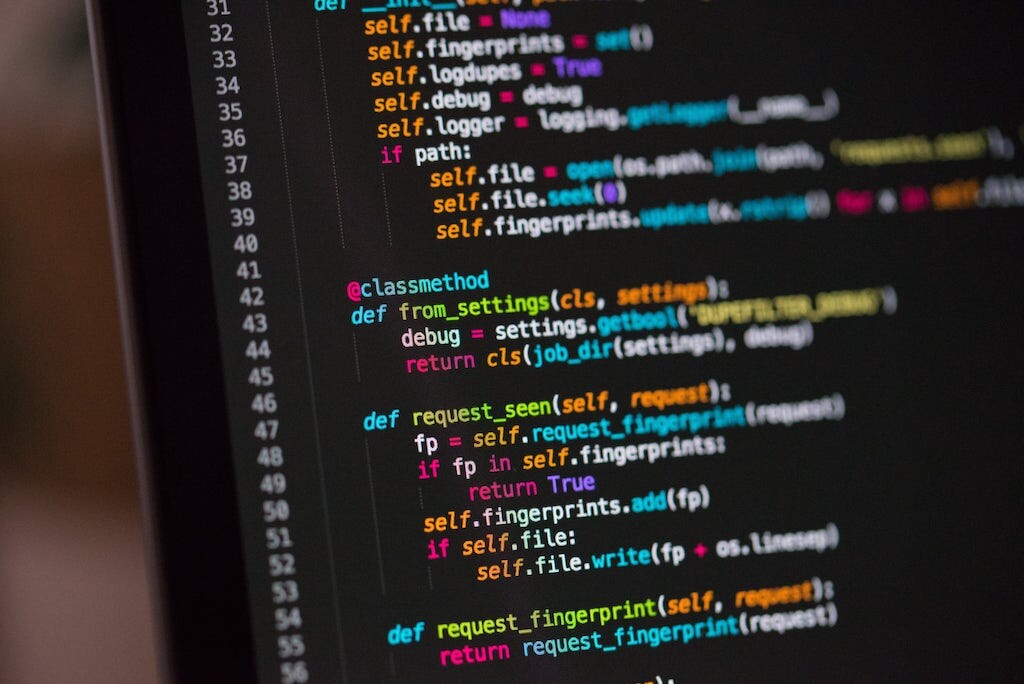
13 Jul Getting started with Symbol in Python – part 2
This post is based on the second in a series published articles from @nobu_kyutech and his work on interacting with the Symbol blockchain using Python. You can find his original examples in Japanese on GitHub
Thanks again @nobu_kyutech!
Retrieving completed transactions for an account
In today’s post I will cover the second of @nobu_kyutech‘s current examples on GitHub which provides a beginners guide to using Python to interact with Symbol API nodes in order to retrieve information.
You may have seen the previous article posted on the blog showing how to write a Python script to retrieve information about a Symbol account address. We will take this one step further today and look at retrieving transactions made by an account.
In this example we will query a node directly using the API and to search confirmed transactions but will do this programmatically in Python. As with the last exercise I will provide a Jupyter notebook that you can use and try to explain @nobu_kyutech‘s code in English (bear with me as I am not a Python programmer!).
Before we start. There are many different types of transaction possible on the Symbol blockchain. Each of these transaction types has a different “enum” code associated with it. These are shown below and explained in more detail here. The important message is that we can use these codes to retrieve only the transactions that we are interested in.
Type of transaction:
0x414C (16716 decimal) - AccountKeyLinkTransaction.
0x4243 (16963 decimal) - VrfKeyLinkTransaction.
0x4143 (16707 decimal) - VotingKeyLinkTransaction.
0x424C (16972 decimal) - NodeKeyLinkTransaction.
0x4141 (16705 decimal) - AggregateCompleteTransaction.
0x4241 (16961 decimal) - AggregateBondedTransaction.
0x414D (16717 decimal) - MosaicDefinitionTransaction.
0x424D (16973 decimal) - MosaicSupplyChangeTransaction.
0x414E (16718 decimal) - NamespaceRegistrationTransaction.
0x424E (16974 decimal) - AddressAliasTransaction.
0x434E (17230 decimal) - MosaicAliasTransaction.
0x4144 (16708 decimal) - AccountMetadataTransaction.
0x4244 (16964 decimal) - MosaicMetadataTransaction.
0x4344 (17220 decimal) - NamespaceMetadataTransaction.
0x4155 (16725 decimal) - MultisigAccountModificationTransaction.
0x4148 (16712 decimal) - HashLockTransaction.
0x4152 (16722 decimal) - SecretLockTransaction.
0x4252 (16978 decimal) - SecretProofTransaction.
0x4150 (16720 decimal) - AccountAddressRestrictionTransaction.
0x4250 (16976 decimal) - AccountMosaicRestrictionTransaction.
0x4350 (17232 decimal) - AccountOperationRestrictionTransaction.
0x4151 (16721 decimal) - MosaicGlobalRestrictionTransaction.
0x4251 (16977 decimal) - MosaicAddressRestrictionTransaction.
0x4154 (16724 decimal) - TransferTransaction.
So let’s get started.. As with the previous example we first need to start a new Jupyter Notebook and the import urllib.request and json modules.
import urllib.request
import json
Then we need to set the node URL (this can be any API node but I have used my own node here). Then we set the account address (this can be any account – e.g. your own!) but I have used the same as in the original GitHub example. Finally we set the enum for the transaction type that we are interested in. In this case we will use TransferTransactions (i.e. transactions that send a token from one account to another).
Set the address of the node that we will connect to for making the query
NODEURL = "http://xymharvesting.net:3000"
Set the account address that we want to query
ADDRESS = "NDLS6GYOIPHATATNAVVOUNJXBD6X4BXU6IRBHIY"
Select the enum value of the transaction type we are interested in (in this case "TransferTransaction")
ENUM_TransferTransaction = 16724
Next we have to get the 48 character address of the account NDLS6GYOIPHATATNAVVOUNJXBD6X4BXU6IRBHIY
as we will need this to fetch the transactions. Why do we need to do this? Well the Symbol API uses this address style so we have to convert from the address format we know and love into a 48 character hexadecimal format.
Get the 48 character address string for the account - this is saved as "ADDRESS48"
req = urllib.request.Request(NODEURL + '/accounts/' + ADDRESS)
with urllib.request.urlopen(req) as res:
accountInfo = json.load(res)
ADDRESS48 = accountInfo['account']['address']
You can see that this gives us a 48 character hexadecimal address string 68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3
corresponding to the account NDLS6GYOIPHATATNAVVOUNJXBD6X4BXU6IRBHIY
:
ADDRESS48
'68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3'
As an aside, you could also perform the same process with the Symbol CLI and would get the same result:
symbol-cli converter base32ToHexAddress -a NDLS6GYOIPHATATNAVVOUNJXBD6X4BXU6IRBHIY
68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3
So we have our address string ADDRESS48
stored and now we can use this to request all confirmed TransferTransactions for this address in descending order (newest first):
# Now we can request confirmed TransferTransactions for this account in descending order (newest first)
# URL would look like this: http://xymharvesting.net:3000/transactions/confirmed?account=68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3&order=desc
url = NODEURL + '/transactions/confirmed'
params = {
'address': ADDRESS,
'order': 'desc',
}
# And print the json format results
req = urllib.request.Request('{}?{}'.format(url, urllib.parse.urlencode(params)))
with urllib.request.urlopen(req) as res:
data = json.load(res)
print(json.dumps(data, indent=2))
This returns the following output:
{
"data": [
{
"meta": {
"height": "336588",
"hash": "86E70E7DE4C9CD4F6970D74633E22C9F58DF9A09ED7DB33E39A5E3D50BD99F03",
"merkleComponentHash": "86E70E7DE4C9CD4F6970D74633E22C9F58DF9A09ED7DB33E39A5E3D50BD99F03",
"index": 1
},
"transaction": {
"size": 17240,
"signature": "FAC3CF1D4A28504201ABD2E6C29E6B1B69DB3F58C672D540C7A073405AE961299A891CA63D6384F734481995700AD0B965B782A465C6708574097E6FD296EB01",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "10196806619",
"transactionsHash": "E9188048F7C8A09AB9CBD203F245C7AA5F933A07934484F8039361E249530708",
"cosignatures": []
},
"id": "60EB71C3C05058331C4F3D05"
},
{
"meta": {
"height": "316353",
"hash": "EDA66C5D8890EA5AAD1E41F287B358A78FD421E46B30E28C6526967C22F4F5BA",
"merkleComponentHash": "EDA66C5D8890EA5AAD1E41F287B358A78FD421E46B30E28C6526967C22F4F5BA",
"index": 0
},
"transaction": {
"size": 14912,
"signature": "76EDCCB402E7A7C5003DEB0D807556A8D67D2F375DABC1CFFE21D2AE111DB3D29F79E37788899F3606BA196779273095AEAF544D2D3A81FE6EAFBB119C415301",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "9589327262",
"transactionsHash": "FB5DB861CCA78769292659B61F553B50F976DFC1F62A79637E9D5B9793466E3C",
"cosignatures": []
},
"id": "60E22CD0C05058331C4D0435"
},
{
"meta": {
"height": "295457",
"hash": "2D0F3D578D53A1ED69BD8BD71A923AAE02E08696DFB4E1B93BADE685D242C9BB",
"merkleComponentHash": "2D0F3D578D53A1ED69BD8BD71A923AAE02E08696DFB4E1B93BADE685D242C9BB",
"index": 3
},
"transaction": {
"size": 18016,
"signature": "0FAE93FE616F020EB00E1D1CDAAD49FCB37CF14EF6D69BF91895495EBACA2733CC481F63F589112DD43B9BB56BCB5A71E61705C3D6F81EA51B90CF4CCB8D6705",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "8961873859",
"transactionsHash": "DB1401359B303E8752CD2EB43A2B81D9FF35EA17C23890AB24936CEA9C38D734",
"cosignatures": []
},
"id": "60D899C1FBD6BD5502064FFA"
},
{
"meta": {
"height": "289930",
"hash": "5E5E82C48E2025D68C23CECAB362B0A18E10D8474DF4C251A6D49F3CF11E9DE9",
"merkleComponentHash": "5E5E82C48E2025D68C23CECAB362B0A18E10D8474DF4C251A6D49F3CF11E9DE9",
"index": 4
},
"transaction": {
"size": 281,
"signature": "88A2FE5EFAE042C3C851B115A0E345CDDC9E82FEF03F3A5CD4F76E66A935CE0438A55977C132D4074286D7B27B2D4E321FAA20C2E3F46D335C4E97EA2BB8690E",
"signerPublicKey": "AD9523A96FFAF2ADF1D2916D0D534A7757C2A782B86CD82E7E3C6C76CB4A1A1A",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "7025",
"deadline": "8795973866",
"recipientAddress": "68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3",
"message": "00F09F9A804D415820424C4F434B532048415256455354494E47204E4F4445F09F92B00AF09F9189302D302D302D302D302D302D302D616E766D652E69392D393930306B2E66756EF09F91880A537570706F72743A207472657A7A6572313240676D61696C2E636F6D",
"mosaics": [
{
"id": "6BED913FA20223F8",
"amount": "1"
}
]
},
"id": "60D611AEFBD6BD550205E712"
},
{
"meta": {
"height": "275403",
"hash": "09B2E987021B9C7F5611583F258CFF3AD302069DC4E51A983ACF4DB62D9A9B0B",
"merkleComponentHash": "09B2E987021B9C7F5611583F258CFF3AD302069DC4E51A983ACF4DB62D9A9B0B",
"index": 1
},
"transaction": {
"size": 14136,
"signature": "79E6168857F8B04F040E2F663F5A5973D5123ACC624B0E1FFD88742DF196DDF55D7F1580F6417C5B23D1F38D2EF64A12A7DDBFA8FAEF48A98BBF0F589B4B530D",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "8359688664",
"transactionsHash": "1150BB99F3BB6CCA6FC43DB960E7222AEACD7750288119793CDF8E98E5FBA0ED",
"cosignatures": []
},
"id": "60CF697F08DD32393C46AF99"
},
{
"meta": {
"height": "259413",
"hash": "CE6C00C60FF0ACCE4EBBE3595BB7431535F9425B8C833387CAA09754D69C2094",
"merkleComponentHash": "CE6C00C60FF0ACCE4EBBE3595BB7431535F9425B8C833387CAA09754D69C2094",
"index": 0
},
"transaction": {
"size": 323,
"signature": "4F3AD3FC82CE85FEF5FB2F70218E72DE1012916B7C6CFA0E4E769BC86EA7B963A3FD4DD006B979B24D70DA8D1E44335C4E5C3B66D99BE5B55F0505E7C58F6D09",
"signerPublicKey": "D6D2E66F470586371B0EBA6B4BE567A75CBDE34D83DE19CA7E0EE948B3F47F76",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "8075",
"deadline": "7879594885",
"recipientAddress": "68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3",
"message": "00E38193E381A1E38289E381AEE8A898E4BA8BE38081E58AA9E3818BE3828AE381BEE38197E3819FEFBC810AE5B091E381AAE3818FE381A6E68190E7B8AEE381A7E38199E3818CE38081E3818AE7A4BCE381BEE381A7E380820A68747470733A2F2F71696974612E636F6D2F6E6F62755F6B7975746563682F6974656D732F6666333863343835303264316265383335333430",
"mosaics": [
{
"id": "6BED913FA20223F8",
"amount": "10000000"
}
]
},
"id": "60C8165FA1EE4B19BC25D409"
},
{
"meta": {
"height": "255132",
"hash": "264C93EB96E0251BD7CBE7451ADC52CC5BD0EF413EE9F4E214D7C4B797F5797C",
"merkleComponentHash": "264C93EB96E0251BD7CBE7451ADC52CC5BD0EF413EE9F4E214D7C4B797F5797C",
"index": 2
},
"transaction": {
"size": 14456,
"signature": "96C4CD4692FECA5067193C0DAEC666747290AA498561DA4383174F05698A00740421A78A125516E229CE92FF07C7180389CA31A38690A166467F4A01E635BF07",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "7751024451",
"transactionsHash": "DD79B5C3D3DC82AA67C131EBC965305435A0833C4E46B3A11A9D084750CE7966",
"cosignatures": []
},
"id": "60C61FF5A1EE4B19BC257344"
},
{
"meta": {
"height": "245658",
"hash": "1E08902B770C45663BE71A2DAD52B8FD86774E3C87E9C8294DE4AA34A34A3AB7",
"merkleComponentHash": "1E08902B770C45663BE71A2DAD52B8FD86774E3C87E9C8294DE4AA34A34A3AB7",
"index": 0
},
"transaction": {
"size": 281,
"signature": "7CEC137F4D9A8F93EF973FAC5C8BA9944CBE50256D918A1E64DE84826586B5BFDEDFAA3BDAFFEB3E4F0070D2D8DA01359AA57C9325F2B05989A5167D5FDD9F03",
"signerPublicKey": "AD9523A96FFAF2ADF1D2916D0D534A7757C2A782B86CD82E7E3C6C76CB4A1A1A",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "7025",
"deadline": "7466527701",
"recipientAddress": "68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3",
"message": "00F09F9A804D415820424C4F434B532048415256455354494E47204E4F4445F09F92B00AF09F9189302D302D302D302D302D302D302D616E766D652E69392D393930306B2E66756EF09F91880A537570706F72743A207472657A7A6572313240676D61696C2E636F6D",
"mosaics": [
{
"id": "6BED913FA20223F8",
"amount": "1"
}
]
},
"id": "60C1C8F1A1EE4B19BC24A250"
},
{
"meta": {
"height": "237515",
"hash": "092D2666941B6C238D9AF4F522D93E8DAE766EB58D875B5C064101A2C95E637D",
"merkleComponentHash": "092D2666941B6C238D9AF4F522D93E8DAE766EB58D875B5C064101A2C95E637D",
"index": 0
},
"transaction": {
"size": 12952,
"signature": "7B99863630E09A28739C210333D43C6461CCD61CDA2A3A8F7AB83663712646861B2C2F55267E3C15788771A8F808275B1068478C68B0719CE72B41B57E784D0B",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "7221932530",
"transactionsHash": "21AFD71BA893F164DA6958F84087166535BD285DB1692EFB9CCC448E70729718",
"cosignatures": []
},
"id": "60BE0D32DECE127478756E32"
},
{
"meta": {
"height": "237495",
"hash": "AABE5C6883FFB5C2EF3B3D4E1A2F7ABDDEA6F348857C47A39B9D02811E636FC5",
"merkleComponentHash": "AABE5C6883FFB5C2EF3B3D4E1A2F7ABDDEA6F348857C47A39B9D02811E636FC5",
"index": 1
},
"transaction": {
"size": 12200,
"signature": "4065C55C3F075A78CF660C4759721E7D18E0FFAAF51BD31E41DFA8290CEC124CF6B4BB2E491F23BAC0FB4E154B5F9D57C5E26D05A04AAE4DAA8BC4713362F304",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "7221315276",
"transactionsHash": "E58E2B25DD445D86BF12C3AC3BEDAB444BB135ADF2F4FD8206CF6CF87A64CC85",
"cosignatures": []
},
"id": "60BE0AB4DECE127478756B69"
},
{
"meta": {
"height": "235032",
"hash": "9DD7B9BDB444A468EDA50570589CEC582E00D7C1FDB535D7F8346D444ED3E583",
"merkleComponentHash": "9DD7B9BDB444A468EDA50570589CEC582E00D7C1FDB535D7F8346D444ED3E583",
"index": 0
},
"transaction": {
"size": 19720,
"signature": "3C4EAB8F8CEFAED92734A3D624519DE49F19CB315DBFC4389858D1B688C7879BF2FC6684C2014BD1A4FB1E77E7689C576D5E2048658CE9AF83552D8B12825A0F",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "7147477464",
"transactionsHash": "4E1F475020E2E54804EFC9C623AB44AC5473F7585B1556640EB2B83652712467",
"cosignatures": []
},
"id": "60BCEA3FDECE127478753E0C"
},
{
"meta": {
"height": "233220",
"hash": "0F85E34D9AF137BE829B90C64AAC31BF96AFB0C90C7CF8C38A54C89EC1298EFE",
"merkleComponentHash": "0F85E34D9AF137BE829B90C64AAC31BF96AFB0C90C7CF8C38A54C89EC1298EFE",
"index": 1
},
"transaction": {
"size": 12328,
"signature": "DFE8C4DF8A1F0D36E73A650352532ED365170D73C1894C859AA8A1DE8C74C387A983A22A807E95E303A3FBDFC40C67D641E4F93BF1883698ACF6BBCD6C24160D",
"signerPublicKey": "6BBE9AF9CCD65F5E438175A8BF0D9AA7C26244679AB99CB1ED83F902662EEC7D",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "2000000",
"deadline": "7093060864",
"transactionsHash": "2C83617C3ECCC3C0F479E07ECBC205B82979B5744F24CB87A5DB9869D89AB562",
"cosignatures": []
},
"id": "60BC15B6DECE127478751508"
},
{
"meta": {
"height": "232952",
"hash": "1743B0886B11386170A0EED9AD2E31877661FBD8F217AE4B46113E5FC9085228",
"merkleComponentHash": "1743B0886B11386170A0EED9AD2E31877661FBD8F217AE4B46113E5FC9085228",
"index": 0
},
"transaction": {
"size": 281,
"signature": "F343CB84EF2DD704B88D596C191566379802F856EF4D26C6B31B45F6D6943667F52B7772531E58F150BBFB5414C0C9D786B412C1F15970C1BBE578081AF73A04",
"signerPublicKey": "AD9523A96FFAF2ADF1D2916D0D534A7757C2A782B86CD82E7E3C6C76CB4A1A1A",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "7025",
"deadline": "7084922895",
"recipientAddress": "68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3",
"message": "00F09F9A804D415820424C4F434B532048415256455354494E47204E4F4445F09F92B00AF09F9189302D302D302D302D302D302D302D616E766D652E69392D393930306B2E66756EF09F91880A537570706F72743A207472657A7A6572313240676D61696C2E636F6D",
"mosaics": [
{
"id": "6BED913FA20223F8",
"amount": "1"
}
]
},
"id": "60BBF691DECE127478750C34"
},
{
"meta": {
"height": "228976",
"hash": "B999F52D421AB5FDC1B8BE5789D03C3E7B670730799F99A1B695A8E303C1B803",
"merkleComponentHash": "B999F52D421AB5FDC1B8BE5789D03C3E7B670730799F99A1B695A8E303C1B803",
"index": 1
},
"transaction": {
"size": 292,
"signature": "319275187DADEF1645A3EB8513A3E3653CEA027518647492A6D162DAE7D79B5DB274C9B3A7576BCA9F1E554B96A488DA1AA75A9B03D50B146A22D57729F2A209",
"signerPublicKey": "5DB471481B3A39AC5CEEE949B64A577C0D5CA67176BE4462145CAD6ABD84CEBC",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "18980",
"deadline": "6965673515",
"recipientAddress": "68DE58C0C5983D74D9819229484C5DC6077951CD2737587C",
"message": "FE2A8061577301E2B07997311C77EA4587DD3723AE7956616DF976E7EC06D97F810D932EF934D212B283CD5D3ACF72FF3297F25CD6E78249AA877E6F90435C9D0366C82CB4F5E3056DA9913FF0AF9A5985893318FF5F4901636498783FA230358BCA20E88CFC4C0C221E0B6DE9359951E01A372DDE193ECCE81A890DCBB497C689C4FB87",
"mosaics": []
},
"id": "60BA2428DECE1274787482CB"
},
{
"meta": {
"height": "228974",
"hash": "C3A0F99CF7862E887EC8942F44A0C2C802F7F859FC5CAFAF8ACFB1EDE05468EB",
"merkleComponentHash": "C3A0F99CF7862E887EC8942F44A0C2C802F7F859FC5CAFAF8ACFB1EDE05468EB",
"index": 3
},
"transaction": {
"size": 256,
"signature": "80DD1C6957BBC910CB985AA034836ED2239DC3E01A113BDFE67FBE41181A744CEFD0609F74FA2C1050B16BC7E2C6942754AC7CC81F7C50C16C6803FB5DD1A107",
"signerPublicKey": "5DB471481B3A39AC5CEEE949B64A577C0D5CA67176BE4462145CAD6ABD84CEBC",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "16640",
"deadline": "6965630622",
"transactionsHash": "468D3D8B81E7F3989D10652167A0592402573837BB0B143815E008377459FFC3",
"cosignatures": []
},
"id": "60BA23F0DECE1274787482B3"
},
{
"meta": {
"height": "219954",
"hash": "A245BDECA6A2FFE548FA0DEC96610188C842EC2DC0F44B485E8CC62088002A5B",
"merkleComponentHash": "A245BDECA6A2FFE548FA0DEC96610188C842EC2DC0F44B485E8CC62088002A5B",
"index": 0
},
"transaction": {
"size": 176,
"signature": "95F6A75A3062DFC0922AA8168A5A5006057225478965F6E8ED3828EC2E15D991D640D121B5135D98BF8AAA6ADF000D818D501F32A474B7084F1B8EFBE96E2904",
"signerPublicKey": "10943DCA976F8C17433F759400B9AF0257D1B36E7D69859B31250039E771004D",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "11440",
"deadline": "6694615121",
"recipientAddress": "68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3",
"mosaics": [
{
"id": "6BED913FA20223F8",
"amount": "2144000000"
}
]
},
"id": "60B6014FDECE127478737493"
},
{
"meta": {
"height": "219951",
"hash": "0FE33AA6C579F7D3D60E5091357A698775FA99FE0BBD261A7D9D736EE87B0C3B",
"merkleComponentHash": "0FE33AA6C579F7D3D60E5091357A698775FA99FE0BBD261A7D9D736EE87B0C3B",
"index": 0
},
"transaction": {
"size": 176,
"signature": "3BB5A1807239C809450280A6A80BB3FD6C80BBAB2799D378A1EDE3788CDB154B7847DC020028DB87B4CCCB59329FE44DBD575AC5D69327F1C28F5203D7460801",
"signerPublicKey": "67AAC9375989D232FC69A17850CB9234BC9726C8B02BED03F64D6C53AE4F219F",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "11440",
"deadline": "6694554513",
"recipientAddress": "68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3",
"mosaics": [
{
"id": "6BED913FA20223F8",
"amount": "8000000000"
}
]
},
"id": "60B6010FDECE12747873748A"
},
{
"meta": {
"height": "188954",
"hash": "853743C7C1014E63261576DA81CFB82CAD15EC823F9B964E7EC1622F5706107C",
"merkleComponentHash": "853743C7C1014E63261576DA81CFB82CAD15EC823F9B964E7EC1622F5706107C",
"index": 0
},
"transaction": {
"size": 176,
"signature": "B1C9FB561C78AE4A93A7B42455D6AAFDA9914FB171B88FA129D13DDF8B134A61AA9C0E6A6D1C66DF479E5DB8837478CFE0F18BC1DE0D71B1A50A33BC1EDF4F0B",
"signerPublicKey": "5DB471481B3A39AC5CEEE949B64A577C0D5CA67176BE4462145CAD6ABD84CEBC",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "50000",
"deadline": "5764020638",
"recipientAddress": "68596CE72E15159BDCEA0FE3B7ECDAC89DC148C8B8B7FA49",
"mosaics": [
{
"id": "6BED913FA20223F8",
"amount": "15400000000"
}
]
},
"id": "60A7CE33DECE12747870B1D3"
},
{
"meta": {
"height": "188805",
"hash": "E12E68D4F126DA2A3C80665F0318B2348AA240CCC126F3A34E4247C06BBAD1AB",
"merkleComponentHash": "E12E68D4F126DA2A3C80665F0318B2348AA240CCC126F3A34E4247C06BBAD1AB",
"index": 0
},
"transaction": {
"size": 256,
"signature": "B56168643EA1414BA76092258A3BFC03A3443AB642692FE27FEAA38E5091FB7EA8CD4452D6A597A7A10518E982E947DBEDEB1821A37D5B4296B2A3BF0FBFD909",
"signerPublicKey": "5DB471481B3A39AC5CEEE949B64A577C0D5CA67176BE4462145CAD6ABD84CEBC",
"version": 1,
"network": 104,
"type": 16705,
"maxFee": "1000000",
"deadline": "5759494621",
"transactionsHash": "034B628BB276065F07CE8C9DA987D629A974110BA2559096D91FE78D24FD968A",
"cosignatures": []
},
"id": "60A7BC82DECE12747870AC19"
},
{
"meta": {
"height": "188326",
"hash": "E47206784E95DFED9CBDD21F766433DED6DC4A686F6FBD6DBED93BF4E489DFDE",
"merkleComponentHash": "E47206784E95DFED9CBDD21F766433DED6DC4A686F6FBD6DBED93BF4E489DFDE",
"index": 1
},
"transaction": {
"size": 176,
"signature": "033FC40F0008B51A97CE724EF994C1497A0AD10573B9D03D384D42882F5AF2EC6181F0B14C2F63C45018A93DBB86E77D163031AA5A8D75C3CDEA930C63F0D102",
"signerPublicKey": "5DB471481B3A39AC5CEEE949B64A577C0D5CA67176BE4462145CAD6ABD84CEBC",
"version": 1,
"network": 104,
"type": 16724,
"maxFee": "50000",
"deadline": "5745032534",
"recipientAddress": "6859ABB7C613FC3CB2D90E215798CA03A094C988A4E14923",
"mosaics": [
{
"id": "6BED913FA20223F8",
"amount": "10000000"
}
]
},
"id": "60A78406DECE127478709281"
}
],
"pagination": {
"pageNumber": 1,
"pageSize": 20
}
}
That’s a lot of output! But actually it is just the first page of most recent transactions. In order to get all transactions you would need to iterate through each page until there are no more entries. This could be done by adding the pageNumber
to the URL and putting this inside a loop. E.g. to get page 2 your URL would look like this:
http://xymharvesting.net:3000/transactions/confirmed?account=68D72F1B0E43CE09826D056AEA353708FD7E06F4F22213A3&order=desc&pageNumber=2
Right, so given all of this information, how do we make sense of this and see how much XYM was transacted in each of the blocks? Here is some code to go through each of the entries in our json results data
.
First we check whether the mosaic sent in the transaction was XYM by comparing the mosaic ID to the XYM mosaic ID 6BED913FA20223F8. If it was then we obtain the amount of XYM that was sent in the transaction. Next we check whether the recipientAddress
in the transaction is the same as our account address which we stored as ADDRESS48
. If this is true then we know that this account received XYM, if it is false, we know that the account sent XYM.
We can print out the block height at the time that the transaction occurred and along with whether our account was the sender or recipient. As we discussed in the previous post the “amount” returned is displayed in microXYM so we have to divide the amount by 1,000,000 to obtain the transaction amount in XYM.
for d in data['data']:
mosaic = None
# Is a mosaic sent or received?
if "mosaics" in d['transaction']:
for xym in d['transaction']['mosaics']:
# is the mosaic ID "6BED913FA20223F8"? (This mosaic ID is XYM)
if xym['id'] == '6BED913FA20223F8':
mosaic = xym['amount']
else:
continue
# Is the account the recipient?
if d['transaction']['recipientAddress'] == ADDRESS48:
isRecipient = True
# If not then it must be the sending account
else:
isRecipient = False
print("height:{}, {}, {}xym".format(d['meta']['height'], 'received' if isRecipient else "sent", int(mosaic)/1000000))
Running this code gives the following result:
height:289930, received, 1e-06xym
height:259413, received, 10.0xym
height:245658, received, 1e-06xym
height:232952, received, 1e-06xym
height:219954, received, 2144.0xym
height:219951, received, 8000.0xym
height:188954, sent, 15400.0xym
height:188326, sent, 10.0xym
Note: some of the amounts are really small and are formatted in scientific notation e.g. 1e-06xym is 0.000001 XYM (1 microXYM). My guess is that this is one of those spammy messages from unscrupulous node owners trying to get you to harvest with them (please don’t do this!).
Great! So we have successfully obtained a list of most recent transactions for an account using Python – we can edit this code however we want, maybe we are interested in a different type of transaction or we want to do this for a list of accounts or obtain all transactions rather than the most recent only. I will let you play around with the code and see what you can do! 😄
You can download the notebook here
Thanks again for reading and a huge thank you to @nobu_kyutech for the original post!
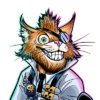
I’m a Symbol and NEM enthusiast and run this blog to try to grow awareness of the platform in the English-speaking world. If you have any Symbol news you would like me to report on or you have an article that you would like to publish then please let me know!
Sorry, the comment form is closed at this time.