
08 May Avatar fun!
Quick post – You probably saw this already but Gimre made a tool for viewing avatars stored as account metadata.
This morning I saw that Anthony had added his avatar to his account so I thought I would do the same and publish some code so that you could give it a go too.
It is quick and dirty but it should do the job π If I remember correctly metadata transactions must be sent as aggregates even if you are writing data to the account that you send the transaction from. Here I am writing data to my own account so I can do it with an aggregate complete transaction. Actually, when I wrote it to my own account NANINEDBABAJKTIKIDKLAMJTWIMMMTHO24LUCKA I had to send it as an aggregate bonded transaction from a different address since the address is a multisig account – if you do the same you’ll need a hasklock transaction which will have to confirm before you send the aggregate bonded tx to bobAddress.
from symbolchain.facade.SymbolFacade import SymbolFacade
from symbolchain.CryptoTypes import PrivateKey
from binascii import unhexlify, hexlify
import http.client
import datetime
import os
import sys
# Read in the PNG file and store the data
png = sys.argv[1]
alicePrivateKey = PrivateKey(unhexlify(sys.argv[2]))
with open(png, 'rb') as file:
png_data = file.read()
facade = SymbolFacade('mainnet')
# Replace with node URL and connection port
node_url = 'xymharvesting.net'
node_port = 3000
# Set mainnet network generation time and then add deadline
netStart = 1615853185
deadline = (int((datetime.datetime.today() + datetime.timedelta(hours=2)).timestamp()) - netStart) * 1000
aliceKeypair = SymbolFacade.KeyPair(alicePrivateKey)
alicePubkey = aliceKeypair.public_key
aliceAddress = facade.network.public_key_to_address(alicePubkey)
# Set up the embedding transaction
embeddedTxs = []
metadataKey = 0x0000524154416541 # Has to have this metadata key for Gimre's tool to read it
metadataValue = png_data
metadata_transaction = facade.transaction_factory.create_embedded({
'type': 'account_metadata_transaction_v1',
'signer_public_key': alicePubkey,
'target_address': aliceAddress,
'scoped_metadata_key': metadataKey,
'value': metadataValue,
'value_size_delta': len(metadataValue),
})
embeddedTxs.append(metadata_transaction)
merkleHash = facade.hash_embedded_transactions(embeddedTxs)
# Set up the aggregate transaction
aggregate_transaction = facade.transaction_factory.create({
'type': 'aggregate_complete_transaction_v2',
'signer_public_key': alicePubkey,
'deadline': deadline,
'transactions_hash': merkleHash,
'transactions': embeddedTxs
})
aggregate_transaction.fee.value = aggregate_transaction.size * 150
# Sign
signature = facade.sign_transaction(aliceKeypair, aggregate_transaction)
tx = facade.transaction_factory.attach_signature(aggregate_transaction, signature)
# Announce the transactions to the network
headers = {'Content-type': 'application/json'}
conn = http.client.HTTPConnection(node_url, node_port)
conn.request("PUT", "/transactions", tx, headers)
response = conn.getresponse()
print(response.status, response.reason)
To run the script you need to have installed the latest Symbol Python SDK (just run pip install symbol-sdk-python
).
Then save the code to file e.g. avatar.py and give it the avatar PNG filename and the account private key as input on the command line:
python avatar.py png_filename.png private_key
NOTE: The avatar should be less than 1KB in size as this is the maximum length of a metadata entry.
NOTE2: Obviously, be careful with your private key – don’t go running this on a public computer!
Check your handwork here: https://gimre-xymcity.github.io/avatar.html
End result:
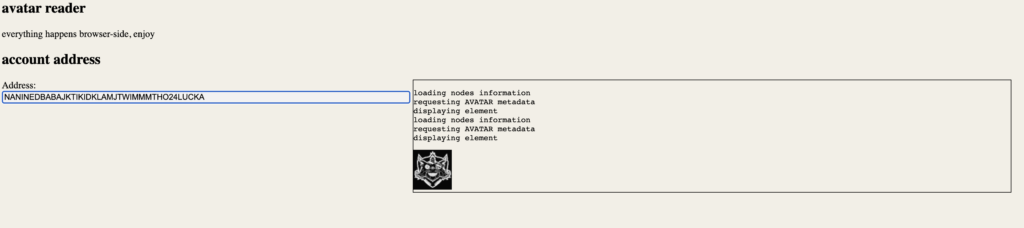
Have fun! π
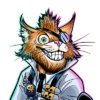
I’m a Symbol and NEM enthusiast and run this blog to try to grow awareness of the platform in the English-speaking world. If you have any Symbol news you would like me to report on or you have an article that you would like to publish then please let me know!
Sorry, the comment form is closed at this time.